 |
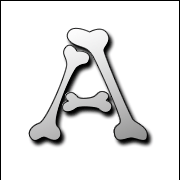













|
 |
 |
 |
ad::Model Class ReferenceMain interface.
More...
#include <model.h>
Collaboration diagram for ad::Model:
[legend]List of all members.
|
Public Types |
enum | Type { STATIC,
DEFORMABLE
} |
| Used to identify if the model is going to be animated or just static.
|
Public Member Functions |
| Model (Type type, UserFunction *texture_loader=0) |
| Initialize the model.
|
void | AllocateRenderPose (ad::Anim *useAnim=0) |
| Allocate the space for the render pose implicitly.
|
void | AllocateRenderPose (unsigned int size) |
| Allocate the space for the render pose directly.
|
void | GenerateRenderPoseMatrices () |
| Generate a world matrix for each bone of the render_pose.
|
int | GenSlot () |
| Create a handle for a binding slot.
|
Mesh * | LoadMesh (std::string filename) |
| Load a mesh and return a handle to it's data.
|
void | BindMesh (Mesh *mesh, int slot=-1) |
| Update the set of visible meshes to include mesh .
|
void | UnBindMesh (Mesh *mesh) |
| Update the set of visible meshes to exclude mesh .
|
void | UnBindMesh (int slot) |
| Update the set of visible meshes to exclude the mesh loaded in slot .
|
AnimPlayer * | AddAnim (std::string filename, float startFrame=0) |
| load an animation (an AnimPlayer blend is created and returned)
|
Blend * | CreateBlend (BlendBase *from=0, BlendBase *to=0) |
| Create a hierarchial blend node.
|
HiBoneMask * | CreateMask (const std::string &selections) |
| Create a selection mask of bones using the hierarchy.
|
virtual void | Update (float timestep) |
| Updates all loaded animations by timestep (in seconds).
|
Public Attributes |
Pose * | renderPose |
| This pose is used to accumulate the results of a blending hierarchy.
|
Matrix * | worldBones |
| This stores the world transformation for each bone of the render pose.
|
Protected Attributes |
std::set< Mesh * > | meshes |
| A model can have several meshes.
|
Model::Type | type |
Anim * | arbitraryAnim |
| An alias to an arbitrary animation (the first one loaded).
|
Detailed Description
Main interface.
This class is meant to simplify the interface between the library and the implementor. - See also:
- ad::Mesh, ad::AnimPlayer
Constructor & Destructor Documentation
|
Initialize the model.
- Parameters:
-
| type | If the model is just a simple non-animated object, use ad::Model::STATIC. If it is animated, use ad::Model::DEFORMABLE |
|
Member Function Documentation
void ad::Model::AllocateRenderPose |
( |
unsigned int |
size |
) |
|
|
|
Allocate the space for the render pose directly.
An alternative to the standard function, which you can use to specify the size of the render pose directly. |
void ad::Model::AllocateRenderPose |
( |
ad::Anim * |
useAnim = 0 |
) |
|
|
|
Allocate the space for the render pose implicitly.
Needed because when constructed, the class doesn't know about any animations and therefore, cannot determine how many bones to make the renderPose. This should be called at some point after loading an animation. If no animation is specified, the first animation that was loaded will be used. |
void ad::Model::BindMesh |
( |
Mesh * |
mesh, |
|
|
int |
slot = -1 |
|
) |
|
|
|
Update the set of visible meshes to include mesh .
Add the mesh to the current list of visible meshes. If a slot handle is provided, this will unbing the mesh previously associated with the slot. |
|
Create a hierarchial blend node.
Creates a blend between two other blends, and returns the instance. Blends don't need to be destroyed and recreated so they stick around for the life of the model. You can reuse a Blend by setting the to and from BlendBases directly. |
HiBoneMask * ad::Model::CreateMask |
( |
const std::string & |
selections |
) |
|
|
|
Create a selection mask of bones using the hierarchy.
Using the naming given for the sample skeleton, it’s very easy to make selection masks using the hierarchy. The way it works is when you select a bone to be either on or off, all of its children inherit that same state unless they are set directly. The color scheme on the figure is: red is set off directly (gray is inherited off), green is set on directly (blue is inherited on). To create this mask then, one would simply need this command:
CreateMask("pelvis 1 spine1 0");
At least one animation should be loaded before using this function. |
void ad::Model::GenerateRenderPoseMatrices |
( |
|
) |
|
|
|
Generate a world matrix for each bone of the render_pose.
Converts the renderPose from local quaternions to world matrices. It is assumed that an animation has already been loadead. |
int ad::Model::GenSlot |
( |
|
) |
|
|
|
Create a handle for a binding slot.
This creates a slot that can only contain one mesh at a time. It is used to automate the process of replacing a mesh on the model with another one. A good example of when you might use this is on a character for an RPG that can wear different clothing and armor. You could model a mesh for severeal types of armor that he could wear on his head, torso, legs, hands and feet. Then when you bind a mesh to this slot, it will automatically hide the mesh already bound to the slot. |
Mesh * ad::Model::LoadMesh |
( |
std::string |
filename |
) |
|
|
|
Load a mesh and return a handle to it's data.
Load a mesh from a file or if it's already been loaded, get the handle for it in memory. |
void ad::Model::UnBindMesh |
( |
Mesh * |
mesh |
) |
|
|
|
Update the set of visible meshes to exclude mesh .
Remove a mesh from the visible list. If the mesh was bound to a slot, this clears the slot. |
void ad::Model::Update |
( |
float |
timestep |
) |
[virtual] |
|
|
Updates all loaded animations by timestep (in seconds).
As it turns out this isn't very useful. It's easier and more efficient to just to call the update for the individual animations in your game code. The best thing to do is just update the animations that are currently effecting the mesh. You can always set the time of the animation directly, so there's no reason to keep animations you're not using running. This function is just offered as a virtual default if you don't write an Update function in the derived class. This could be useful for a model that just plays the same animation the whole time. |
The documentation for this class was generated from the following files:
|
 |